The Live Bee Hive
A Prisma Arduino Project
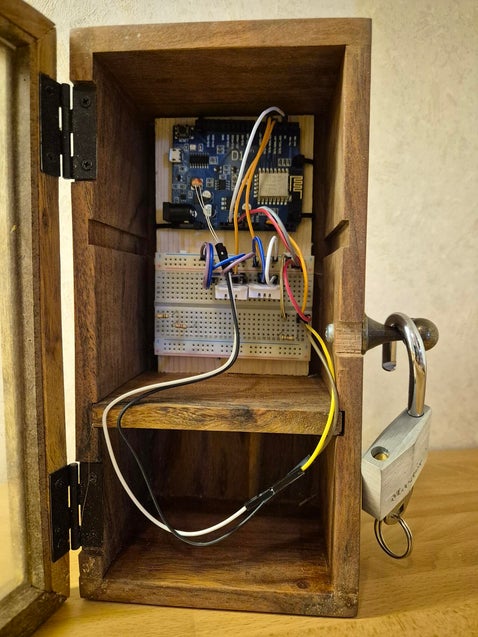
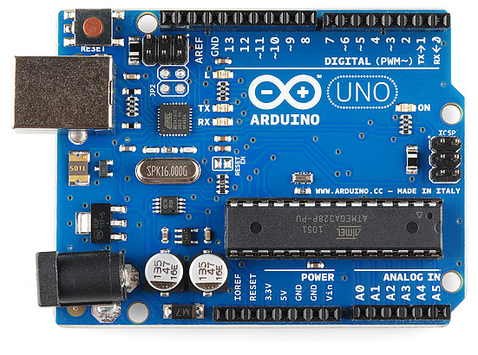
<= Prototyping the Arduino
We have built our own Beehive weather station. It monitors the temperature and moisture inside the hive as well as the temperature and moisture in the garden. It also measures the sunshine daylight. With a infrared interruptor we "count" (by proxy) the amount of bees that fly to and from the hive. The weight of the hive is measured since in winter it is a sign of honey consumption rate and in summer it shows the honey increase (which beekeeper call Honey Flow). The data is sent via wifi to the internet and online you can see the Hives condition.
Right now the station is off for maintenance but when it is running again you can see the live data at:
We will show on this site the full design of the project and we hope it stimulates you to develop your own Arduino, or Internet Of Things (IOT) projects. Here below the Arduino code currently in use, but as said we will explain more later.....
#include <ESP8266WiFi.h>
#include <ThingSpeak.h>
#include <DHT.h>
#include <HX711.h>
// WiFi and ThingSpeak credentials
const char* ssid = "YourSSID";
const char* password = "YourPassword";
unsigned long myChannelNumber = YOUR_CHANNEL_ID;
const char* myWriteAPIKey = "YOUR_API_KEY";
// Sensor pins
#define LIGHT_SENSOR_PIN A0
#define DHT_INNER_PIN D1
#define DHT_OUTER_PIN D2
#define DHT_TYPE DHT22
#define HX711_DOUT_PIN D3
#define HX711_SCK_PIN D4
#define IR_LED_PIN D5
#define IR_RECEIVER_PIN D6
#define BATTERY_VOLTAGE_PIN A0
// Initialize sensors
DHT dhtInner(DHT_INNER_PIN, DHT_TYPE);
DHT dhtOuter(DHT_OUTER_PIN, DHT_TYPE);
HX711 scale;
WiFiClient client;
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
ThingSpeak.begin(client);
dhtInner.begin();
dhtOuter.begin();
scale.begin(HX711_DOUT_PIN, HX711_SCK_PIN);
}
void loop() {
// Measure light intensity
int lightIntensity = analogRead(LIGHT_SENSOR_PIN);
// Measure inner hive temperature and humidity
float innerTemp = dhtInner.readTemperature();
float innerHumidity = dhtInner.readHumidity();
// Measure outer hive temperature and humidity
float outerTemp = dhtOuter.readTemperature();
float outerHumidity = dhtOuter.readHumidity();
// Measure hive weight
float hiveWeight = scale.get_units(10);
// Count bees using IR sensors
int beeCount = measureBees(10);
// Measure battery voltage
float batteryVoltage = analogRead(BATTERY_VOLTAGE_PIN) * (12.0 / 1024.0);
// Send data to ThingSpeak
ThingSpeak.setField(1, lightIntensity);
ThingSpeak.setField(2, innerTemp);
ThingSpeak.setField(3, innerHumidity);
ThingSpeak.setField(4, outerTemp);
ThingSpeak.setField(5, outerHumidity);
ThingSpeak.setField(6, hiveWeight);
ThingSpeak.setField(7, beeCount);
ThingSpeak.setField(8, batteryVoltage);
ThingSpeak.writeFields(myChannelNumber, myWriteAPIKey);
// Deep sleep for 1 hour
ESP.deepSleep(3600e6);
}
int measureBees(int duration) {
int count = 0;
unsigned long startTime = millis();
while (millis() - startTime < duration * 1000) {
if (digitalRead(IR_RECEIVER_PIN) == LOW) {
count++;
delay(10);
}
}
return count;
}
Create Your Own Website With Webador